Merhabalar ben pygame ile bir oyun yapmaya çalışıyorum. Birkaç gün öncesine kadar işlem yapabiliyorken bilgisayarıma parça taktırdığımda bu hatayı almaya başladım ve sadece bu dosyada değil diğer dosyalarda da aynı hatayı alıyorum. Yardım ederseniz sevinirim.
Not: Bu kodlara uzun süredir bakmadım bazı noktaları hatırlayamayabilirim. Kod ve görseller aşağıda bulunmaktadır.
Kod:
import pygame, sys
import random
import ctypes
myappid = 'mycompany.myproduct.subproduct.version'
ctypes.windll.shell32.SetCurrentProcessExplicitAppUserModelID(myappid)
pygame.init()
# ekran ayarları
width = 900
heigh = 600
path = "Zindanlar ve Zindanlar V2.5/"
icon = pygame.image.load(path + "assets/icon.png")
main_win = pygame.display.set_mode((width,heigh))
pygame.display.set_caption("Zindanlar ve Zindanlar V2.5")
pygame.display.set_icon(icon)
# gömülü sınıflar
class TextButton():
def __init__(self, x, y, text, fsize = 20, csize = 2, renk = (255,255,255), x_ayar = 3, y_ayar = 4, uzunluk_ayar = 6, yukseklik_ayar = 5):
font = pygame.font.SysFont("calibri", fsize)
self.text = font.render(text, True, renk)
self.rect = self.text.get_rect()
self.rect_width = self.text.get_width()
self.rect_heigh = self.text.get_height()
self.rect.topleft = (x, y)
self.cerceve_x = x - x_ayar
self.cerceve_y = y - y_ayar
self.cerceve_kalınlık = csize
self.cerceve_uzunluk = uzunluk_ayar
self.cerceve_yukseklik = yukseklik_ayar
self.clicked = False
def draw(self, surface):
action = False
#get mouse position
pos = pygame.mouse.get_pos()
#check mouseover and clicked conditions
if self.rect.collidepoint(pos):
if pygame.mouse.get_pressed()[0] == 1 and self.clicked == False:
self.clicked = True
action = True
if pygame.mouse.get_pressed()[0] == 0:
self.clicked = False
#draw button on screen
surface.blit(self.text, (self.rect.x, self.rect.y))
self.cerceve = pygame.draw.rect(main_win, (255,255,255), (self.cerceve_x, self.cerceve_y, self.rect_width + self.cerceve_uzunluk, self.rect_heigh + self.cerceve_yukseklik), self.cerceve_kalınlık)
return action
class ImageButton():
def __init__(self, x, y, image, scale):
width = image.get_width()
height = image.get_height()
self.image = pygame.transform.scale(image, (int(width * scale), int(height * scale)))
self.rect = self.image.get_rect()
self.rect.topleft = (x, y)
self.clicked = False
def draw(self, surface):
action = False
#get mouse position
pos = pygame.mouse.get_pos()
#check mouseover and clicked conditions
if self.rect.collidepoint(pos):
if pygame.mouse.get_pressed()[0] == 1 and self.clicked == False:
self.clicked = True
action = True
if pygame.mouse.get_pressed()[0] == 0:
self.clicked = False
#draw button on screen
surface.blit(self.image, (self.rect.x, self.rect.y))
return action
# gömülü fonksiyonlar
def yaz(text, x, y, renk, size = 15, font = "calibri"):
font = pygame.font.SysFont(font, size)
yazi = font.render(text, True, renk)
main_win.blit(yazi, (x, y))
# global değişkenler
clock = pygame.time.Clock()
mode = "starter_mode"
# resimler
exit_key_pict = pygame.image.load(path + "assets/exit_pict.png")
settings_key_pict = pygame.image.load(path + "assets/settings_pict.png")
starter_menu_pict = pygame.image.load(path + "assets/starter_menu.jpg")
starter_menu_pict = pygame.transform.scale(starter_menu_pict, (width, heigh))
main_menu_pict = pygame.image.load(path + "assets/main_world.png")
main_menu_pict = pygame.transform.scale(main_menu_pict, (width, heigh))
# düğmeler
exit_key = TextButton(10,435,"Çıkış", fsize = 25, csize = 3)
new_game_key = TextButton(10,260,"Yeni", fsize = 25, csize = 3)
load_game_key = TextButton(10,295,"Yükle", fsize = 25, csize = 3)
guide_key = TextButton(10, 330, "Rehber", fsize = 25, csize = 3)
settings_key = TextButton(10,365,"Ayarlar", fsize = 25, csize = 3)
dev_key = TextButton(10, 400, "Geliştirici", fsize = 25, csize = 3)
bolge1_key = TextButton(317, 270, "o", 37, -1, (132,43,54))
bolge2_key = TextButton(411, 306, "o", 37, -1, (137,145,93))
bolge3_key = TextButton(525, 379, "o", 37, -1, (169,124,56))
# döngü
loop = True
while loop:
position = pygame.mouse.get_pos()
for event in pygame.event.get():
if event.type == pygame.QUIT:
loop = False
if event.type == pygame.KEYDOWN:
if mode == "starter_mode":
mode = "main_mode"
if mode == "starter_mode":
main_win.blit(starter_menu_pict, (0,0))
# tuş kontrol
if exit_key.draw(main_win):
loop = False
if new_game_key.draw(main_win):
mode = "main_mode"
if load_game_key.draw(main_win):
print("Yapılmadı")
if guide_key.draw(main_win):
print("oldu 3")
if settings_key.draw(main_win):
print("oldu 4")
if dev_key.draw(main_win):
print("oldu 5")
if mode == "main_mode":
main_win.blit(main_menu_pict, (0,0))
if bolge1_key.draw(main_win):
print("giriş yapıldı")
if bolge2_key.draw(main_win):
print("giriş yapıldı")
if bolge3_key.draw(main_win):
print("giriş yapıldı")
if pygame.mouse.get_pressed()[0]:
print(position)
pygame.display.update()
clock.tick(60)
pygame.quit()
sys.exit()
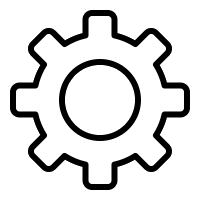

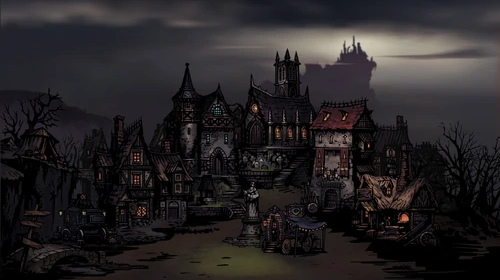